Data Aggregation
In this exercise we build an application designed to aggregate text messages sent to recipients. We would like to store all messages sent to single user in unique aggregate.
For the input topic, we send messages in the following format: ${timestamp}#${sender}#${receiver}#${message}, for example:
- key: null, value: 1728148429#john#maria#Hello how are you?
- key: null, value: 1728148430#maria#john#I am good, thank you
- key: null, value: 1728148431#maria#john#And how are you?
- key: null, value: 1728148450#john#maria#I am good too
- key: null, value: 1728148450#john#maria#I'd like to talk with you about Apache Kafka training scheduled next week
On the output topic, we would like to have number of messages sent to single receiver, like:
- key: maria, value: { "messages": [ { "senderTime": 1728148429, "inboxTime": 1728148459, "sender": "john", "message": "Hello how are you?"}]
- key: john, value: { "messages": [ { "senderTime": 1728148430, "inboxTime": 1728148450, "sender": "maria", "message": "I am good, thank you"}]
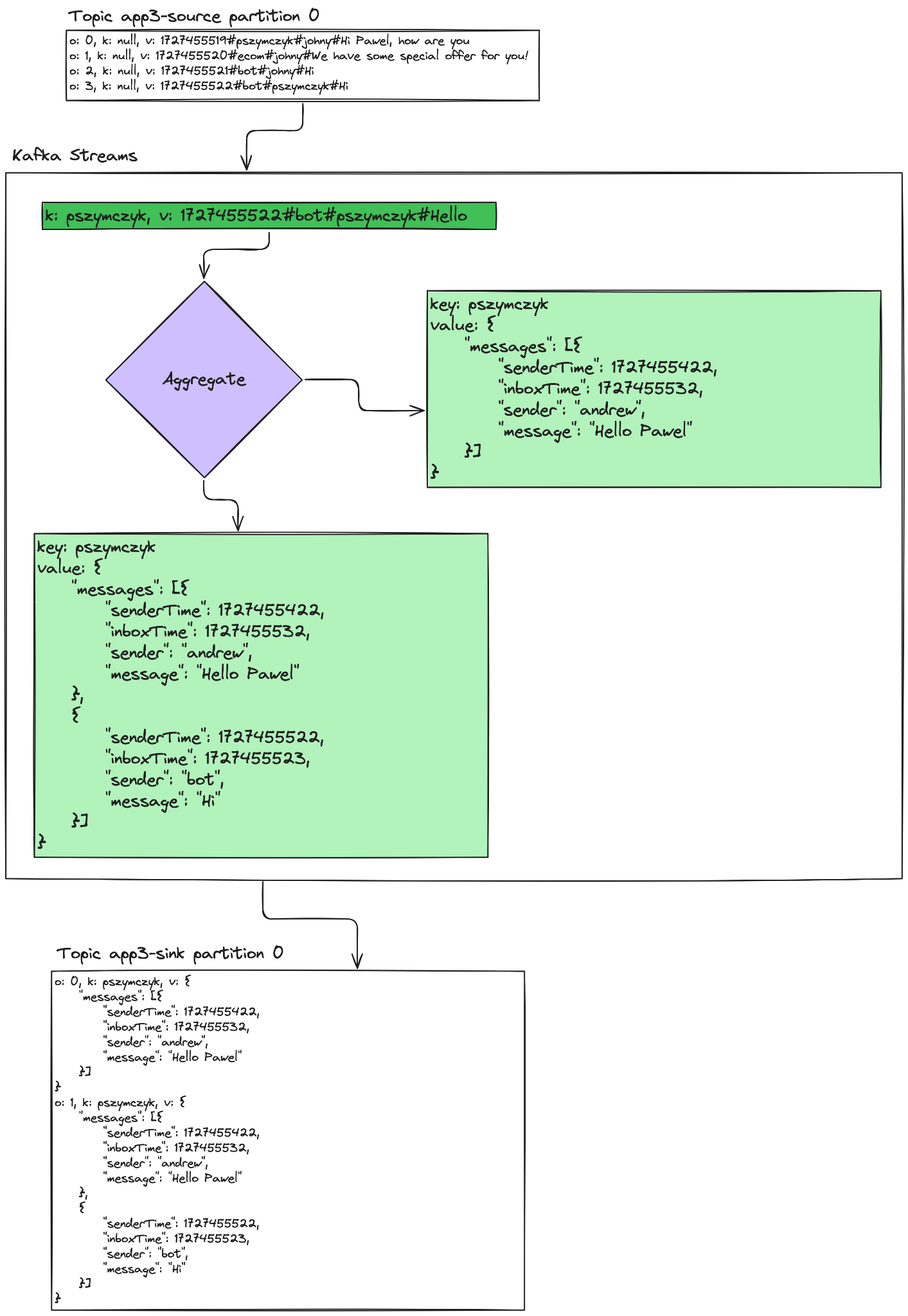
Implementation
- Clone repository github.com/pszymczyk/kafka-streams-playground.
- Checkout to the app3 branch.
- In the solution you should use classes provided in com.pszymczyk.common package - Inbox, InboxMessage, JsonSerde.
- Open InboxApp.java file.
- Go to buildKafkaStreamsTopology() method. You can find there basic StreamsBuilder setup, we will use it to define our application topology.
- Let's start from consuming messages from app3-source topic, use stream(...) method.
- In the next step we need to change message key from null to message receiver, use selectKey(...) method.
- Now we need to group together messages with the same key, use groupByKey() method.
- When the messages are grouped together, we can aggregate them by evaluating aggregate(...) method. You should pass exactly three arguments there:
- new Initializer()
- new Aggregator<String, Message, Inbox>()
- Materialized.with(Serdes.String(), JsonSerdes.newSerdes(Inbox.class))
- new Initializer()
- Result of aggregation should be KTable<String, Inbox>.
- When aggregation is done, last thing we need to do is to forward computation results to sink topic. We should utilize methods toStream() and to() now.
- Run tests and check solution correctness.