Custom deserializer
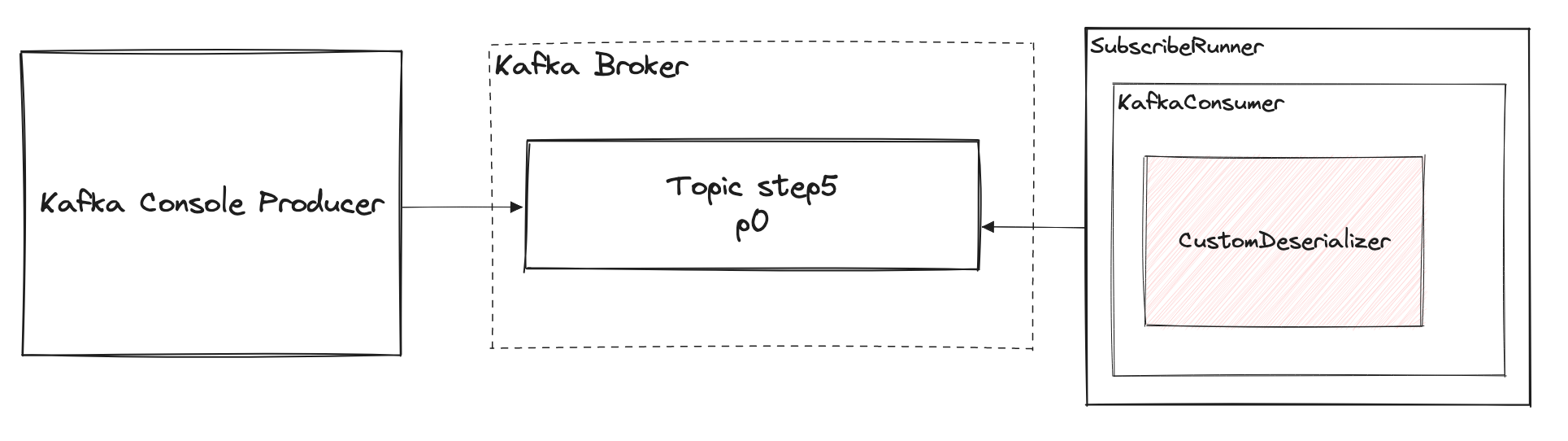
In this exercise, participants will implement a custom record deserializer using the mechanisms provided by the Apache Kafka native driver (https://kafka.apache.org/21/ja...). The goal is to deserialize data from one of the most widely used data formats—JSON—into a simple Data Transfer Object (DTO) in Java.
Objectives:
- Understand Kafka Deserialization: Familiarize yourself with the deserialization process in Kafka and the role of custom deserializers.
- Implement the Deserializer: Create a custom deserializer that accurately converts JSON data into a Java DTO class.
- Testing and Validation: Ensure that the deserialization process is thoroughly tested and that the resulting DTO accurately represents the original data.
This exercise aims to enhance your understanding of data processing in Kafka and improve your skills in working with JSON and Java DTOs.
Implementation
- Clone repository https://github.com/pszymczyk/kafka-native-java-playground
- Switch to step5 branch
- In the SubscribeRunner class, there is a main(...) method that starts consuming messages from step5 Topic
- Implement messages deserialization - from JSON to object of class Customer.
- Use provided CustomDeserializer class
- Send the correct JSON, eg. { "name": "janek", "age": "72"} to step5 Topic
- When the solution is correct, a message should be displayed on the application logs
- Send some incorrect JSON, eg. { "asd": "qwww }
- Check application behaviour