Partitioner and Producer Interceptor
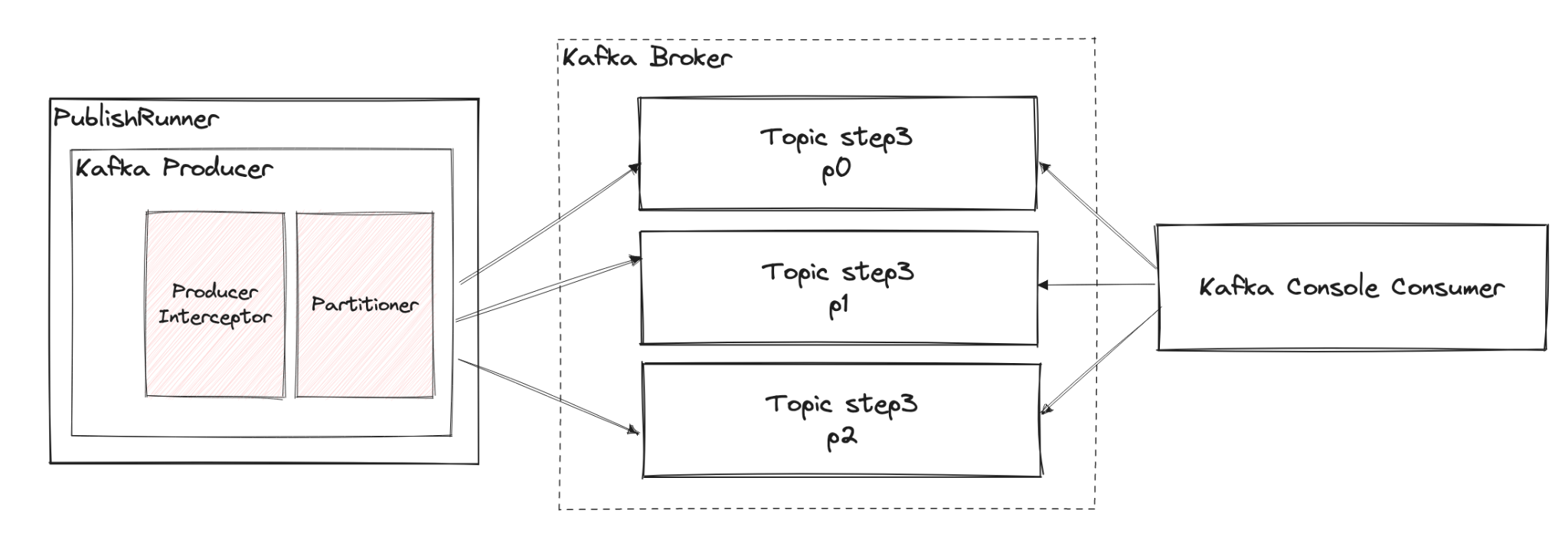
In this task, we will implement a custom Partitioner for a Kafka Producer, as well as a Producer Interceptor. The objective is to enhance the functionality of the Kafka Producer by creating a tailored approach to message distribution and interception.
Objectives:
- Custom Partitioner:
- Develop a custom Partitioner that determines how messages are distributed across Kafka partitions. This should consider factors such as message key, value, or other relevant criteria to optimize load balancing and message processing.
- Producer Interceptor:
- Implement a Producer Interceptor that allows for pre-processing and post-processing of messages sent by the producer. This could include tasks such as logging, metrics collection, or modifying the message before it is sent to Kafka.
Implementation
- Clone repository https://github.com/pszymczyk/kafka-native-java-playground
- Switch to step3 branch
- In the MetadataEnrichmentInterceptor class, implement your own interceptor that adds two headers to the message
- local_time, with the value of the current time in the Canada/Yukon zone
- source, with the name of the microservice producing the message, i.e. Step3_microservice
- In the PositiveNegativePartitioner class, implement your own partitioning mechanism working according to the following rules:
- If the value of the sent message is 0, send the message to partition number 0
- If the value of the sent message is a number less than zero, send the message to partition number 1
- If the value of the sent message is a number greater than zero, send the message to partition number 2
- In the PublishRunner class, register the interceptor and the partitioner
- Run PublishRunner, every 100ms messages from the range [-100,100] are sent to the topic step3
- Run Kafka Console Consumer and check the operation of the partitioner and interceptor
- ./kafka-console-consumer.sh --topic step3 --bootstrap-server localhost:9092 --property print.partition=true --property print.headers=true